MutableProSharedFlow – Enhanced SharedFlow with Initial Value in Kotlin
An open-source Kotlin class that combines the features of MutableSharedFlow and StateFlow
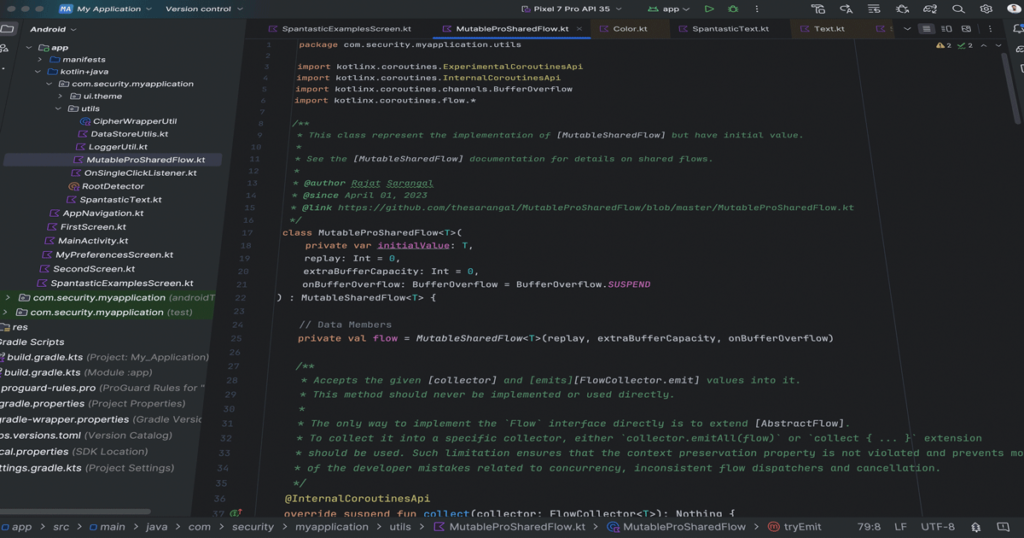
Introduction
This is a Kotlin class implementation of [MutableSharedFlow] with an initial value. Shared flows are a type of flow that allows multiple collectors to receive the same stream of data. This implementation is called MutableProSharedFlow and extends the MutableSharedFlow class. It allows the user to set an initial value when creating the flow. Actually this class is mixer of MutableSharedFlow and StateFlow.
Features
Initial Value Support – Provides an initial value to collectors, similar to StateFlow
.
Multiple Collectors – Allows multiple collectors to receive the same data stream simultaneously.
Configurable Parameters – Offers customization of replay count, extra buffer capacity, and buffer overflow strategy.
Lightweight and Efficient – Designed for optimal performance with minimal overhead.
How to use
// Create an instance of MutableProSharedFlow with an initial value
val sharedFlow = MutableProSharedFlow<Int>(initialValue = 0)
// Collector 1
launch {
sharedFlow.collect { value ->
println("Collector 1 received: $value")
}
}
// Collector 2
launch {
sharedFlow.collect { value ->
println("Collector 2 received: $value")
}
}
// Emit new values
sharedFlow.emit(1)
sharedFlow.emit(2)
// In this example, both collectors receive the initial value 0 upon
// subscription and subsequently receive the emitted values 1 and 2.
Parameters
The class takes in the following parameters:
initialValue
: the initial value of the flowreplay
: the number of values that will be buffered and replayed to new collectors when they start collectingextraBufferCapacity
: additional buffer capacity for buffering values that can be collected lateronBufferOverflow
: the strategy to use when the buffer overflows, the default strategy is to suspend
The class has the following functions:
collect
: accepts aFlowCollector
and emits values to it. If the replay cache is empty, the initial value is emitted first.emit
: emits a value to the shared flow and updates the initial valueresetReplayCache
: resets the replay cache to an empty statetryEmit
: tries to emit a value to the shared flow without suspending. If it succeeds, it updates the initial valuesubscriptionCount
: returns the number of subscribers to the shared flowreplayCache
: returns a snapshot of the replay cache Thecollect
,emit
,resetReplayCache
, andtryEmit
functions are overridden from theMutableSharedFlow
class. ThesubscriptionCount
andreplayCache
functions are deprecated in theMutableSharedFlow
class and are therefore overridden in this implementation.
Benefits
- Value will be collect even if new value is same as last value. [Property of SharedFlow]
- Flow has initial value [Property of StateFlow]. By use of getValue() method.
Crafted In
Let’s Improve Together
Your reactions guide development. Please share your thoughts on new features or recommendations. Unitedly we can improve to help all.