SpantasticLibrary – Effortless Text Styling for Android
An open-source Kotlin library for applying custom spans on TextView text
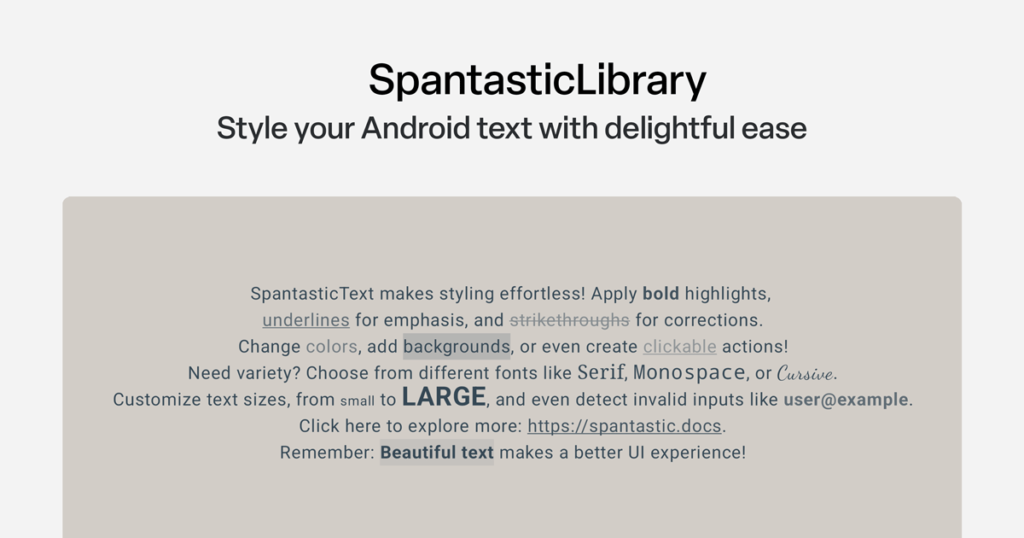
Introduction
Spantastic Library is a lightweight Android library for applying custom spans on text in TextView. It provides an easy way to apply custom styles, fonts, colors, and click events to specific parts of the text in a TextView.
Features
Customizable Spans – Apply custom fonts, colors, styles, and click events to specific parts of the text.
Easy Integration – Seamlessly integrates into existing Android projects with minimal setup.
Lightweight – Designed to be efficient without adding significant overhead to your application.
How to use
Just Show Underline under specific word
// Java
String sentence = "Spannable with single word underline.";
TextView tvOne = findViewById(R.id.tv_one);
new Spantastic.Builder(tvOne, sentence)
.setSpan("underline")
.showUnderline(true)
.apply();
// Kotlin
var sentence = "Spannable with single word underline."
val tvOne = findViewById<TextView>(R.id.tv_one)
Spantastic.Builder(tvOne, sentence)
.setSpan("underline")
.showUnderline(true)
.apply()
Show Underline and make Text BOLD for Multiple words
// Java
String sentence = "Spannable with underline and bold for multiple words.";
List<String> strings = new ArrayList<>();
strings.add("spannable");
strings.add("underline");
strings.add("bold");
TextView tvTwo = findViewById(R.id.tv_two);
new Spantastic.Builder(tvTwo, sentence)
.setSpanList(strings)
.showUnderline(true)
.setTypeface(Typeface.create(Typeface.DEFAULT, Typeface.BOLD))
.apply();
// Kotlin
sentence = "Spannable with underline and bold for multiple words."
var strings: ArrayList<String> = ArrayList()
strings.add("spannable")
strings.add("underline")
strings.add("bold")
val tvTwo = findViewById<TextView>(R.id.tv_two)
Spantastic.Builder(tvTwo, sentence)
.setSpanList(strings)
.showUnderline(true)
.setTypeface(Typeface.create(Typeface.DEFAULT, Typeface.BOLD))
.apply()
Set Click on Multiple Words with Bold Style
// Java
String sentence = "Spannable with click, color, underline and bold for multiple words.";
List<String> strings = new ArrayList<>();
strings.add("click");
strings.add("color");
strings.add("underline");
strings.add("bold");
TextView tvThree = findViewById(R.id.tv_three);
new Spantastic.Builder(tvThree, sentence)
.setSpanList(strings)
.showUnderline(true)
.setSpanColor(ContextCompat.getColor(this, R.color.colorAccent))
.setTypeface(Typeface.create(Typeface.DEFAULT, Typeface.BOLD))
.setClickCallback(new Spantastic.SpannableCallBack() {
@Override
public void onSpanClick(String spanString, Object... object) {
Toast.makeText(MainActivity.this, String.format(Locale.ENGLISH,
"\"%s\" Word Clicked", spanString), Toast.LENGTH_SHORT)
.show();
}
})
.apply();
// Kotlin
sentence =
"Spannable with click, color, underline and bold for multiple words."
strings = ArrayList()
strings.add("click")
strings.add("color")
strings.add("underline")
strings.add("bold")
val tvThree = findViewById<TextView>(R.id.tv_three)
Spantastic.Builder(tvThree, sentence)
.setSpanList(strings)
.showUnderline(true)
.setSpanColor(ContextCompat.getColor(this, R.color.colorAccent))
.setTypeface(Typeface.create(Typeface.DEFAULT, Typeface.BOLD))
.setClickCallback(object : Spantastic.SpannableCallBack {
override fun onSpanClick(spanString: String?, vararg `object`: Any?) {
Toast.makeText(
this@KotlinActivity, String.format(
Locale.ENGLISH,
"\"%s\" Word Clicked", spanString
), Toast.LENGTH_SHORT
)
.show()
}
})
.apply()
Change TextSizeSet Click on Multiple Words with Bold Style
// Java
String sentence = "Spantastic with custom text size for words.";
TextView tvFour = findViewById(R.id.tv_four);
new Spantastic.Builder(tvFour, sentence)
.setSpan("text size")
.setTextSize(TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 20,
this.getResources().getDisplayMetrics()))
.apply();
// Kotlin
sentence = "Spantastic with custom text size for words."
val tvFour = findViewById<TextView>(R.id.tv_four)
Spantastic.Builder(tvFour, sentence)
.setSpan("text size")
.setTextSize(
TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 20f,
this.resources.displayMetrics
)
)
.apply()
sentence = “Spantastic with custom styles for multiple words.”;
// Java
TextView tvFive = findViewById(R.id.tv_five);
List<SpanModel> spanModelList = new ArrayList<>();
spanModelList.add(new SpanModel("custom", ContextCompat.getColor(this, R.color.colorPrimary), "custom", true, Typeface.create(Typeface.DEFAULT, Typeface.NORMAL), TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 15, this.getResources().getDisplayMetrics())));
spanModelList.add(new SpanModel("styles", ContextCompat.getColor(this, R.color.colorPrimaryDark), "styles", false, Typeface.create(Typeface.DEFAULT, Typeface.BOLD), TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 10, this.getResources().getDisplayMetrics())));
spanModelList.add(new SpanModel("for", ContextCompat.getColor(this, R.color.colorAccent), "for", true, Typeface.create(Typeface.SERIF, Typeface.ITALIC), TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 18, this.getResources().getDisplayMetrics())));
spanModelList.add(new SpanModel("multiple", Color.parseColor("#FFFF5722"), "multiple", null, Typeface.create(Typeface.MONOSPACE, Typeface.BOLD_ITALIC), TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 13, this.getResources().getDisplayMetrics())));
spanModelList.add(new SpanModel("words", Color.parseColor("#FF9C27B0"), "words", true, Typeface.create(Typeface.SANS_SERIF, Typeface.BOLD), null));
new Spantastic.Builder(tvFive, sentence)
.setCustomSpanModel(spanModelList)
.setClickCallback(new Spantastic.SpannableCallBack() {
@Override
public void onSpanClick(String spanString, Object... object) {
Toast.makeText(MainActivity.this,
String.format(Locale.ENGLISH, "\"%s\" Word Clicked", spanString),
Toast.LENGTH_SHORT).show();
}
})
.apply();
// Kotlin
sentence = "Spantastic with custom styles for multiple words."
val tvFive = findViewById<TextView>(R.id.tv_five)
val spanModelList: MutableList<SpanModel> = ArrayList()
spanModelList.add(
SpanModel(
"custom",
ContextCompat.getColor(this, R.color.colorPrimary), "custom",
true, Typeface.create(Typeface.DEFAULT, Typeface.NORMAL),
TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 15f,
this.resources.displayMetrics
)
)
)
spanModelList.add(
SpanModel(
"styles",
ContextCompat.getColor(this, R.color.colorPrimaryDark), "styles",
false, Typeface.create(Typeface.DEFAULT, Typeface.BOLD),
TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 10f,
this.resources.displayMetrics
)
)
)
spanModelList.add(
SpanModel(
"for",
ContextCompat.getColor(this, R.color.colorAccent), "for",
true, Typeface.create(Typeface.SERIF, Typeface.ITALIC),
TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 18f,
this.resources.displayMetrics
)
)
)
spanModelList.add(
SpanModel(
"multiple",
Color.parseColor("#FFFF5722"), "multiple", null,
Typeface.create(Typeface.MONOSPACE, Typeface.BOLD_ITALIC),
TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_SP, 13f,
this.resources.displayMetrics
)
)
)
spanModelList.add(
SpanModel(
"words",
Color.parseColor("#FF9C27B0"), "words", true,
Typeface.create(Typeface.SANS_SERIF, Typeface.BOLD), null
)
)
Spantastic.Builder(tvFive, sentence)
.setCustomSpanModel(spanModelList)
.setClickCallback(object : Spantastic.SpannableCallBack {
override fun onSpanClick(spanString: String?, vararg `object`: Any?) {
Toast.makeText(
this@KotlinActivity, String.format(
Locale.ENGLISH, "\"%s\" Word Clicked", spanString
),
Toast.LENGTH_SHORT
).show()
}
})
Above Example’s Output
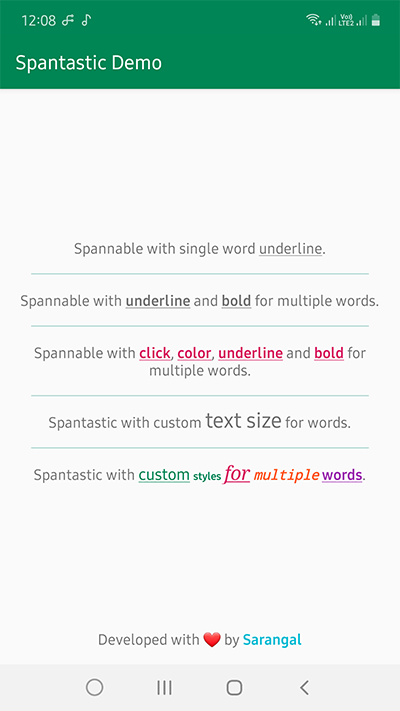
Crafted In
Let’s Improve Together
Your reactions guide development. Please share your thoughts on new features or recommendations. Unitedly we can improve to help all.