SpantasticText – Easy Text Styling with Spans in Jetpack Compose
An open-source Kotlin library providing a composable function to create styled and interactive text spans in Jetpack Compose
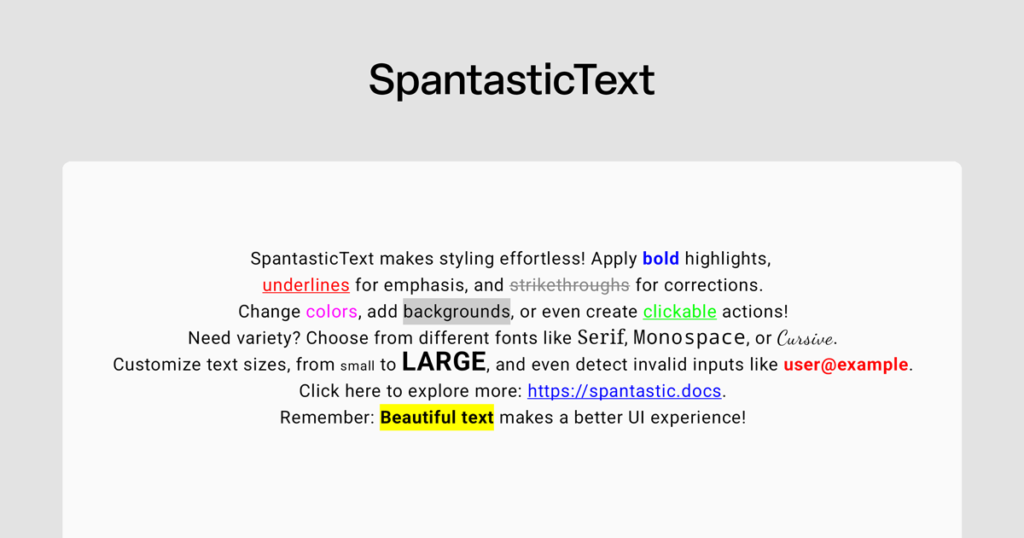
Introduction
SpantasticText is a Jetpack Compose utility that simplifies the creation of styled and clickable text spans within your Android applications. By leveraging this composable function, developers can easily apply various text styles and handle click events, enhancing the interactivity and visual appeal of their app’s user interface.
Features
Customizable Text Styles – Apply different fonts, colors, sizes, and other text styles to specific parts of your text.
Clickable Spans – Define clickable areas within your text to handle user interactions seamlessly.
Composable Function – Integrates smoothly with Jetpack Compose, adhering to modern Android UI development practices.
Lightweight and Efficient – Designed for optimal performance with minimal overhead.
Example
SpantasticText(
text = """
SpantasticText makes styling effortless! Apply bold highlights,
underlines for emphasis, and strikethroughs for corrections.
Change colors, add backgrounds, or even create clickable actions!
Need variety? Choose from different fonts like Serif, Monospace, or Cursive.
Customize text sizes, from small to LARGE, and even detect invalid inputs like user@example.
Click here to explore more: https://spantastic.docs.
Remember: Beautiful text makes a better UI experience!
""".trimIndent(),
spanModels = listOf(
// Bold Text
SpanModel(
spanString = "bold",
color = Color.Blue,
fontWeight = FontWeight.Bold
),
// Underlined Text
SpanModel(
spanString = "underlines",
color = Color.Red,
showUnderline = true
),
// Strikethrough Text
SpanModel(
spanString = "strikethroughs",
color = Color.Gray,
showStrikeThrough = true
),
// Color Text
SpanModel(
spanString = "colors",
color = Color.Magenta
),
// Background Color Text
SpanModel(
spanString = "backgrounds",
backgroundColor = Color.LightGray
),
// Clickable Action
SpanModel(
spanString = "clickable",
color = Color.Green,
showUnderline = true,
callbackKey = "click_action"
),
// Font Variations
SpanModel(
spanString = "Serif",
fontFamily = FontFamily.Serif,
fontSize = 18f
),
SpanModel(
spanString = "Monospace",
fontFamily = FontFamily.Monospace,
fontSize = 18f
),
SpanModel(
spanString = "Cursive",
fontFamily = FontFamily.Cursive,
fontSize = 18f
),
// Text Size Variations
SpanModel(
spanString = "small",
fontSize = 12f
),
SpanModel(
spanString = "LARGE",
fontSize = 24f,
fontWeight = FontWeight.Bold
),
// Highlighting Invalid Input
SpanModel(
spanString = "user@example",
color = Color.Red,
fontWeight = FontWeight.Bold
),
// Clickable URL
SpanModel(
spanString = "https://spantastic.docs",
color = Color.Blue,
showUnderline = true,
callbackKey = "docs_url"
),
// Important Reminder
SpanModel(
spanString = "Beautiful text",
backgroundColor = Color.Yellow,
fontWeight = FontWeight.Bold
)
),
onClick = { key ->
when (key) {
"click_action" -> Toast.makeText(context, "Performing a clickable action!", Toast.LENGTH_SHORT).show()
"docs_url" -> Toast.makeText(context, "Opening SpantasticText documentation...", Toast.LENGTH_SHORT).show()
}
}
)
Output
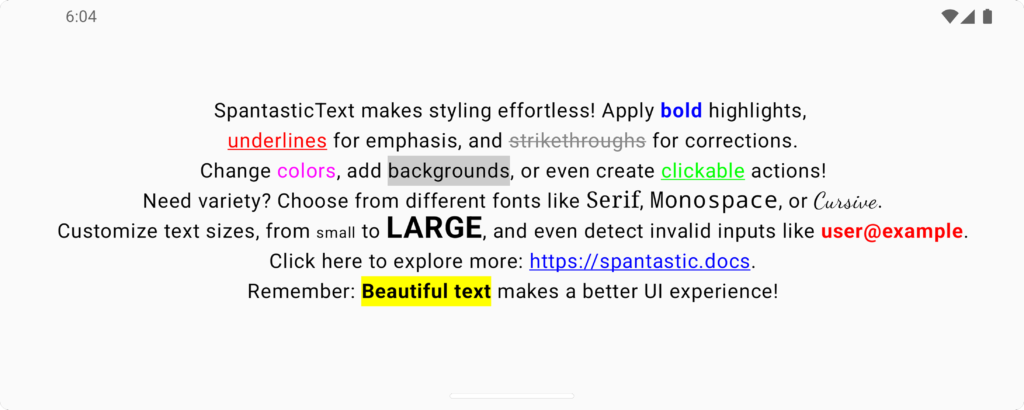
Crafted In
Let’s Improve Together
Your reactions guide development. Please share your thoughts on new features or recommendations. Unitedly we can improve to help all.